About this chapter
This chapter describes how to write code that allows you to access and change a graph in your application at runtime.
In PowerBuilder, there are two ways to display graphs:
-
In a DataWindow, using data retrieved from the DataWindow data source
-
In a graph control in a window or user object, using data supplied by your application code
This chapter discusses the graph control and describes how your application code can supply data for the graph and manipulate its appearance.
For information about graphs in DataWindows, see DataWindow Programmers Guide and DataWindow Reference.
To learn about designing graphs and setting graph properties in the painters, see the section called “Working with Graphs” in Users Guide.
Graph controls in a window can be enabled or disabled, visible or invisible, and can be used in drag and drop. You can also write code that uses events of graph controls and additional graph functions.
Properties of graph controls
You can access (and optionally modify) a graph by addressing its properties in code at runtime. There are two kinds of graph properties:
-
Properties of the graph definition itself
These properties are initially set in the painter when you create a graph. They include a graph's type, title, axis labels, whether axes have major divisions, and so on. For 3D graphs, this includes the Render 3D property that uses transparency rather than overlays to enhance a graph's appearance and give it a more sophisticated look.
-
Properties of the data
These properties are relevant only at runtime, when data has been loaded into the graph. They include the number of series in a graph (series are created at runtime), colors of bars or columns for a series, whether the series is an overlay, text that identifies the categories (categories are created at runtime), and so on.
Events of graph controls
Graph controls have the events listed in the following table.
Clicked |
DragLeave |
Constructor |
DragWithin |
Destructor |
GetFocus |
DoubleClicked |
LoseFocus |
DragDrop |
Other |
DragEnter |
RButtonDown |
So, for example, you can write a script that is invoked when a user clicks a graph or drags an object on a graph (as long as the graph is enabled).
Functions for graph controls
You use the PowerScript graph functions in the following table to manipulate data in a graph.
Function |
Action |
---|---|
AddCategory |
Adds a category |
AddData |
Adds a data point |
AddSeries |
Adds a series |
DeleteCategory |
Deletes a category |
DeleteData |
Deletes a data point |
DeleteSeries |
Deletes a series |
ImportClipboard |
Copies data from the clipboard to a graph |
ImportFile |
Copies the data in a text file to a graph |
ImportString |
Copies the contents of a string to a graph |
InsertCategory |
Inserts a category before another category |
InsertData |
Inserts a data point before another data point in a series |
InsertSeries |
Inserts a series before another series |
ModifyData |
Changes the value of a data point |
Reset |
Resets the graph's data |
This section shows how you can populate an empty graph with data.
Using AddSeries
You use AddSeries to create a series. AddSeries has this syntax:
graphName.AddSeries ( seriesName )
AddSeries returns an integer that identifies the series that was created. The first series is numbered 1, the second is 2, and so on. Typically you use this number as the first argument in other graph functions that manipulate the series.
So to create a series named Stellar, code:
int SNum SNum = gr_1.AddSeries("Stellar")
Using AddData
You use AddData to add data points to a specified series. AddData has this syntax:
graphName.AddData ( seriesNumber, value, categoryLabel )
The first argument to AddData is the number assigned by PowerBuilder to the series. So to add two data points to the Stellar series, whose number is stored by the variable SNum (as shown above), code:
gr_1.AddData(SNum, 12, "Q1") // Category is Q1 gr_1.AddData(SNum, 14, "Q2") // Category is Q2
Getting a series number
You can use the FindSeries function to determine the number PowerBuilder has assigned to a series. FindSeries returns the series number. This is useful when you write general-purpose functions to manipulate graphs.
An example
Say you want to graph quarterly printer sales. Here is a script that populates the graph with data:
gr_1.Reset( All! ) // Resets the graph. // Create first series and populate with data. int SNum SNum = gr_1.AddSeries("Stellar") gr_1.AddData(SNum, 12, "Q1") // Category is Q1. gr_1.AddData(SNum, 14, "Q2") // Category is Q2. gr_1.Adddata(SNum, 18, "Q3") // Category is Q3. gr_1.AddData(SNum, 25, "Q4") // Category is Q4. // Create second series and populate with data. SNum = gr_1.AddSeries("Cosmic") // Use the same categories as for series 1 so the data // appears next to the series 1 data. gr_1.AddData(SNum, 18, "Q1") gr_1.AddData(SNum, 24, "Q2") gr_1.Adddata(SNum, 38, "Q3") gr_1.AddData(SNum, 45, "Q4") // Create third series and populate with data. SNum = gr_1.AddSeries("Galactic") gr_1.AddData(SNum, 44, "Q1") gr_1.AddData(SNum, 44, "Q2") gr_1.Adddata(SNum, 58, "Q3") gr_1.AddData(SNum, 65, "Q4")
Here is the resulting graph:
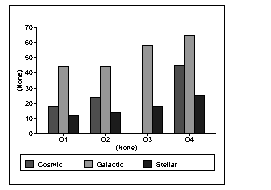
You can add, modify, and delete data in a graph in a window through graph functions anytime during execution.
For more information
For complete information about each graph function, see PowerScript Reference.
When you define a graph in the Window or User Object painter, you specify its behavior and appearance. For example, you might define a graph as a column graph with a certain title, divide its Value axis into four major divisions, and so on. Each of these entries corresponds to a property of a graph. For example, all graphs have an enumerated attribute GraphType, which specifies the type of graph.
When dynamically changing the graph type
If you change the graph type, be sure to change other properties as needed to define the new graph properly.
You can change these graph properties at runtime by assigning values to the graph's properties in scripts. For example, to change the type of the graph gr_emp to Column, you could code:
gr_emp.GraphType = ColGraph!
To change the title of the graph at runtime, you could code:
gr_emp.Title = "New title"
Graphs consist of parts: a title, a legend, and axes. Each of these parts has a set of display properties. These display properties are themselves stored as properties in a subobject (structure) of Graph called grDispAttr.
For example, graphs have a Title property, which specifies the title's text. Graphs also have a property TitleDispAttr, of type grDispAttr, which itself contains properties that specify all the characteristics of the title text, such as the font, size, whether the text is italicized, and so on.
Similarly, graphs have axes, each of which also has a set of properties. These properties are stored in a subobject (structure) of Graph called grAxis. For example, graphs have a property Values of type grAxis, which contains properties that specify the Value axis's properties, such as whether to use autoscaling of values, the number of major and minor divisions, the axis label, and so on.
Here is a representation of the properties of a graph:
Graph int Height int Depth grGraphType GraphType boolean Border string Title grDispAttr TitleDispAttr, LegendDispAttr, PieDispAttr string FaceName int TextSize boolean Italic grAxis Values, Category, Series boolean AutoScale int MajorDivisions int MinorDivisions string Label
You use dot notation to reference these display properties. For example, one of the properties of a graph's title is whether the text is italicized or not. That information is stored in the boolean Italic property in the TitleDispAttr property of the graph.
For example, to italicize title of graph gr_emp, code:
gr_emp.TitleDispAttr.Italic = TRUE
Similarly, to turn on autoscaling of a graph's Values axis, code:
gr_emp.Values.Autoscale = TRUE
To change the label text for the Values axis, code:
gr_emp.Values.Label = "New label"
To change the alignment of the label text in the Values axis, code:
gr_emp.Values.LabelDispAttr.Alignment = Left!
For a complete list of graph properties, see Objects and Controls or use the Browser.
For more about the Browser, see the section called “Browsing the class hierarchy” in Users Guide.
To access properties related to a graph's data during execution, you use PowerScript graph functions. The graph functions related to data fall into several categories:
-
Functions that provide information about a graph's data
-
Functions that save data from a graph
-
Functions that change the color, fill patterns, and other visual properties of data
How to use the functions
To call functions for a graph in a graph control, use the following syntax:
graphControlName.FunctionName ( Arguments )
For example, to get a count of the categories in the window graph gr_printer, code:
Ccount = gr_printer.CategoryCount()
Different syntax for graphs in DataWindows
The syntax for the same functions is more complex when the graph is in a DataWindow, like this:
DataWindowName.FunctionName ( "graphName", otherArguments... )
For more information, see the section called “Manipulating Graphs” in DataWindow Programmers Guide.
The PowerScript functions in the following table allow you to get information about data in a graph at runtime.
Function |
Information provided |
---|---|
CategoryCount |
The number of categories in a graph |
CategoryName |
The name of a category, given its number |
DataCount |
The number of data points in a series |
FindCategory |
The number of a category, given its name |
FindSeries |
The number of a series, given its name |
GetData |
The value of a data point, given its series and position (superseded by GetDataValue, which is more flexible) |
GetDataLabelling |
Indicates whether the data at a given data point is labeled in a DirectX 3D graph |
GetDataieExplode |
The percentage by which a pie slice is exploded |
GetDataStyle |
The color, fill pattern, or other visual property of a specified data point |
GetDataTransparency |
Indicates the transparency value of a given data point in a DirectX 3D graph |
GetDataValue |
The value of a data point, given its series and position |
GetSeriesLabelling |
Indicates whether a data series has a label in a DirectX 3D graph |
GetSeriesStyle |
The color, fill pattern, or other visual property of a specified series |
GetSeriesTransparency |
Indicates the transparency value of a data series in a DirectX 3D graph |
ObjectAtPointer |
The graph element over which the mouse was positioned when it was clicked |
SeriesCount |
The number of series in a graph |
SeriesName |
The name of a series, given its number |
The PowerScript functions in the following table allow you to save data from the graph.
Function |
Action |
---|---|
Clipboard |
Copies a bitmap image of the specified graph to the clipboard |
SaveAs |
Saves the data in the underlying graph to the clipboard or to a file in one of a number of formats |
The PowerScript functions in the following table allow you to modify the appearance of data in a graph.
Function |
Action |
---|---|
ResetDataColors |
Resets the color for a specific data point |
SetDataLabelling |
Sets the label for a data point in a DirectX 3D graph |
SetDataPieExplode |
Explodes a slice in a pie graph |
SetDataStyle |
Sets the color, fill pattern, or other visual property for a specific data point |
SetDataTransparency |
Sets the transparency value for a data point in a DirectX 3D graph |
SetSeriesLabelling |
Sets the label for a series in a DirectX 3D graph |
SetSeriesStyle |
Sets the color, fill pattern, or other visual property for a series |
SetSeriesTransparency |
Sets the transparency value for a series in a DirectX 3D graph |
Users can click graphs during execution. PowerScript provides a function called ObjectAtPointer that stores information about what was clicked. You can use this function in a number of ways in Clicked scripts. For example, you can provide the user with the ability to point and click on a data value in a graph and see information about the value in a message box. This section shows you how.
Clicked events and graphs
To cause actions when a user clicks a graph, you write a Clicked script for the graph control. The control must be enabled. Otherwise, the Clicked event does not occur.
Using ObjectAtPointer
ObjectAtPointer has the following syntax.
graphName.ObjectAtPointer ( seriesNumber, dataNumber )
You should call ObjectAtPointer in the first statement of a Clicked script.
When called, ObjectAtPointer does three things:
-
It returns the kind of object clicked on as a grObjectType enumerated value. For example, if the user clicks on a data point, ObjectAtPointer returns TypeData!. If the user clicks on the graph's title, ObjectAtPointer returns TypeTitle!.
For a complete list of the enumerated values of grObjectType, open the Browser and click the Enumerated tab.
-
It stores the number of the series the pointer was over in the variable seriesNumber, which is an argument passed by reference.
-
It stores the number of the data point in the variable dataNumber, also an argument passed by reference.
After you have the series and data point numbers, you can use other graph functions to get or provide information. For example, you might want to report to the user the value of the clicked data point.
Example
Assume there is a graph gr_sale in a window. The following script for its Clicked event displays a message box:
-
If the user clicks on a series (that is, if ObjectAtPointer returns TypeSeries!), the message box shows the name of the series clicked on. The script uses the function SeriesName to get the series name, given the series number stored by ObjectAtPointer.
-
If the user clicks on a data point (that is, if ObjectAtPointer returns TypeData!), the message box lists the name of the series and the value clicked on. The script uses GetData to get the data's value, given the data's series and data point number:
int SeriesNum, DataNum double Value grObjectType ObjectType string SeriesName, ValueAsString // The following function stores the series number // clicked on in SeriesNum and stores the number // of the data point clicked on in DataNum. ObjectType = & gr_sale.ObjectAtPointer (SeriesNum, DataNum) IF ObjectType = TypeSeries! THEN SeriesName = gr_sale.SeriesName (SeriesNum) MessageBox("Graph", & "You clicked on the series " + SeriesName) ELSEIF ObjectType = TypeData! THEN Value = gr_sale. GetData (SeriesNum, DataNum) ValueAsString = String(Value) MessageBox("Graph", & gr_sale. SeriesName (SeriesNum) + & " value is " + ValueAsString) END IF